In one of my last posts, I explain how to call an Azure Function to create a basic dynamic Hello World Logic App, but it was a simple introduction to Azure Functions Integration inside Logic Apps. However, there are many other scenarios that weren’t addressed in the post like:
- How can you pass inputs to an Azure Function?
- How to deal with “complex” outputs from the Azure Function?
- What types of Azure Functions are supported?
- and so on.
In this post, we will try to deepen some of these topics and provide you some tips and tricks to get you started using Azure Functions on your Logic Apps
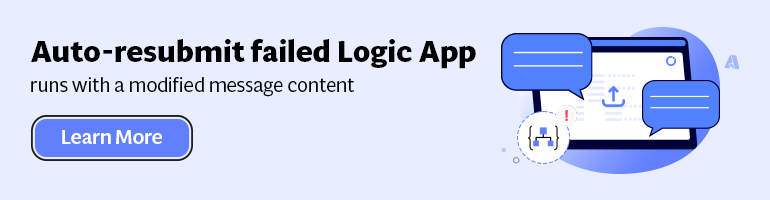
What types of Azure Functions are supported?
Azure Functions provides you a variety of templates options to create a function, from BlobTrigger (C# and Node), Empty (C# and Node), EventHubTrigger (C# and Node), HttpTrigger (C# and Node)… to Generic WebHook (C# and Node)

Despite all this varied offer, at the moment (everything on Azure can evolve and change rapidly), the only supported Azure Functions that you can call inside your Logic Apps are:
- WebHook Node JS Functions
- Or WebHook C# Functions

How can we pass inputs to an Azure Function and access to the output?
After a while is very simple to understand what is need to do to send inputs to an Azure Function, however, access to the response output, or outputs, and use them in subsequent connectors… can bring some challenges.
Let’s create some basic samples in order to try to demystify some of these challenges, and for these samples, we will be using WebHook Node JS Functions.
An important thing you need to be aware of is that, within Logic Apps, you need to always send a valid JSON message as input to a Webbook Function.
Sending empty inputs to an Azure Function
So for example, if your Function doesn’t really need to have any input, you will still need to send a valid JSON message, but because any value that we spend is unnecessary, the trick here is to send an empty JSON message:
- “{}” – without double quotes

If we don’t do this and leave the Input Payload Object empty or “” the Logic App will fail with the following error:
“The WebHook request must contain an entity body formatted as JSON.”
Sending inputs to an Azure Function
To send inputs to the Azure Function we need to, for example, manually create our JSON message as on the “Input payload object” property inside the Configure Function Inputs panel. Let’s look at an example:
- I add a “Twitter – When a new tweet appears” trigger in my Logic App, that looks for a new tweet containing the hashtag “#LogicApps”
- In my case, I will use “#nodejs” for the sake of speed, since they are constantly emerging tweets with this hashtag

And I want to send the “Tweeted by” and “Tweet text” as inputs for my Azure Functions, let’s ignore for now what the function does, but the function needs to receive the following JSON format.
{ "Tweet": { "by": "New York", "text": "21 2nd Street" } }
To accomplish this on the “INPUT PAYLOAD OBJECT” property I need, for example (that depends on the code of the function), to:
- On the text property, type: {“Tweet”: {“by”:
- Here I’m starting to create my JSON message
- Next, I need to select the “Tweeted by” output from my twitter trigger, you can see all the elements of previous steps that you can use on the bottom of the window of this action.

- After that we need to continue to create the remainder of the message by typing:
- ,”text”:
- Select the “Tweeted text” output from the twitter trigger and finally type the rest of the JSON message:
- }}
The end result will look like this:

Retrieving the output of an Azure function
Now that we know how to send inputs to our Azure Function, let’s see how can use their output in subsequent connectors.
Following the input sample provided above:
{ "Tweet": { "by": "New York", "text": "21 2nd Street" } }
Let’s say that we have the following Azure Function:
module.exports = function (context, data) { var Tweet = data.Tweet; var d = new Date().getTime(); var uuid = 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) { var r = (d + Math.random()*16)%16 | 0; d = Math.floor(d/16); return (c=='x' ? r : (r&0x3|0x8)).toString(16); }); // Response of the function to be used later. context.res = { body: { Msg: 'Sandro you have a new tweet msg: ' + Tweet.text, FileName: 'TweetMsg_' + uuid + ".txt" } }; context.done(); };
That returns the following output (in the result of the input provided earlier):
{ "Msg": "Sandro you have a new tweet msg: This is #LogicApps demo msg", "FileName": "TweetMsg_315381a5-847f-4962-9f1f-4fb65a958753.txt" }
And we want to create a Dropbox file containing only the value of the output parameter “Msg” and the name of the file should be the value of the output parameter “Filename”.
But for now, let’s set the filename statically as “Demo1.txt”
If we add a “Dropbox – Create file” connector to our Logic App

- Set the Folder path, let’s say the root by typing: /
- Note: That you can also use the “…” button to navigate to your Dropbox folders
- Click on the Filename and type: Demo1.txt
- And then click on “File content” text box, we will notice that the output parameters of the Azure Function are in design “Body” and “Status code”.
- Let’s select “Body” as the content of the file we want to create.

If we save and execute the Logic App when a new tweet is found, we will see a new file in our Dropbox, however, the content of the file will be the entire JSON message that is returned by the function

We can also receive the following error on the following Logic App executions, we will deal with it shortly:
“body”: {
“status”: 409,
“message”: “File ‘/Demo1.txt’ already exists. Use the Update operation to update an existing file.”,
“source”: “127.0.0.1”
}
So how I can access to the output parameters and using them on the Dropbox connector properties?
The trick here is:
- at design time, set the function output parameter “Body” both in the “FILE NAME” and “FILE CONTENT” properties of the Dropbox connector (or any other connector)
- You don’t need to do this step, but the reason why I do it’s because I am a little lazy and I want the designer to do half of the work for me

- And then on the top of the Logic Apps Designer click in “Code view” option, for us to edit these values in “Code View” mode
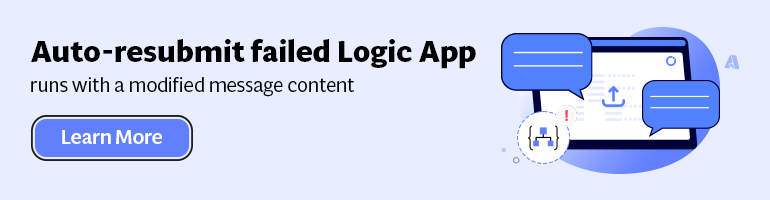

- Then we need to edit Create file inputs to have the expected values

- Change the “inputs” –> “queries” –> “name” property to: @{body(‘TwitterPassDataToFunction’)[‘FileName’]
- And the “inputs” –> ”body” to: @{body(‘TwitterPassDataToFunction’)[‘Msg’]

Now if we change to the Logic Apps Designer, by clicking “Designer” option, we will notice that the designer already renders these properties to set the expected parameters. Parameters that are not there out-of-the-box

If we once again save and execute the Logic App, when a new tweet is found, we will see a new file in our Dropbox with the expected file name:

And if we check the content of the file(s), we will also see that the file has the expected content:

I hope that the next version will have a better experience to deal with the Azure Functions outputs to have, by default, a first-class experience.
Azure Function returning only one parameter
The previous sample returns two parameters, now if we want to return only one parameter, creating a concept of “a string variable” that you can use in subsequent connectors. We can make small changes to our Azure Function to have a first-class experience without the need to switch to “Code View” to change the inputs of the connector.
Using the same sample, let’s assume that:
- Our function only returns the message that we want to put in the context of our file
- we want the file name to have the “Tweet id” as the filename, instead of the previous “Filename” that will no longer exist
- The file context will be provided by the output of the Azure Function
If we create a new Azure Function from the Logic App Designer it will suggest the following code, that of course, we can change:
module.exports = function (context, data) { var Tweet = data.Tweet; // Response of the function to be used later. context.res = { body: { greeting: 'Hello ' + Tweet + '!' } }; context.done(); };
If we use this template approach and change the code to have, for example:
body: { Msg: 'Sandro you have a new tweet msg: ' + Tweet.text }
We will face the same problem as the previous sample, and we would have to access to “Code View” and manually change/set the parameters of the subsequent connectors.
But in this case, we can implement a different approach to have a better user experience in the Logic Apps Designer. Instead of following the suggested approach we can implement the following code:
module.exports = function (context, data) { var Tweet = data.Tweet; // Response of the function to be used later. context.res = { body: 'Sandro you have a new tweet msg: ' + Tweet.text }; context.done(); };
If we try to execute this function through the Azure Portal, we will notice that the output is no longer a JSON message:
{ "Msg": "Sandro you have a new tweet msg: This is #LogicApps demo msg", "FileName": "TweetMsg_83557388-97c4-4503-a3cd-7be145f31d42.txt" }
But instead is now a string:
Sandro you have a new tweet msg: 21 2nd Street

If we now go back to the logic app that we created earlier and:
- Set the “Dropbox – Create file” filename property with the Twitter output parameter “Tweet id” and add a file extension by typing “.txt”
- And the file content with the Azure Function output parameters: “Body”

If we, once again, save and execute the Logic App, when a new tweet is found, we will see a new file(s) in our Dropbox are created with the expected file name:
And if we, once again, check the content of the file(s), we will also see that the file has the expected content:

Having this way, a better user experience inside the Logic Apps Designer. However, this approach only works if the output is a single value (int, string, double).
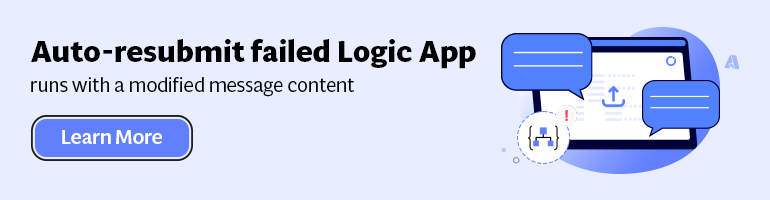
Great article about how to use Azure Function in Logic Apps. Looking forward to see some real world integration scenario use case with Azure Function.
if i need to put all the tweets in one text file what should we do ?
Hi Sandro,
I need your help. I have a one stored procedure on azure sql db. I have logic app , it will capture new data load into that table. After triggering I want to call Azure Function . This azure function will call SP.I tried it but azure function require “REQUEST BOSY”. How do I fill this.